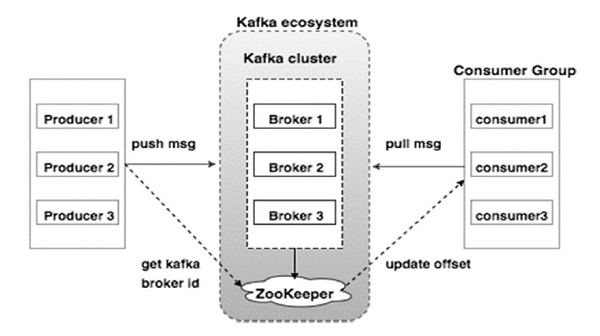
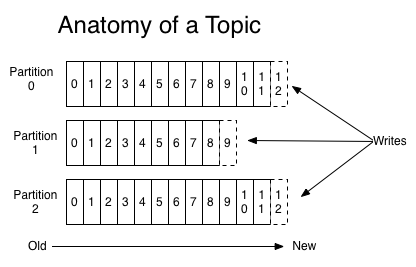
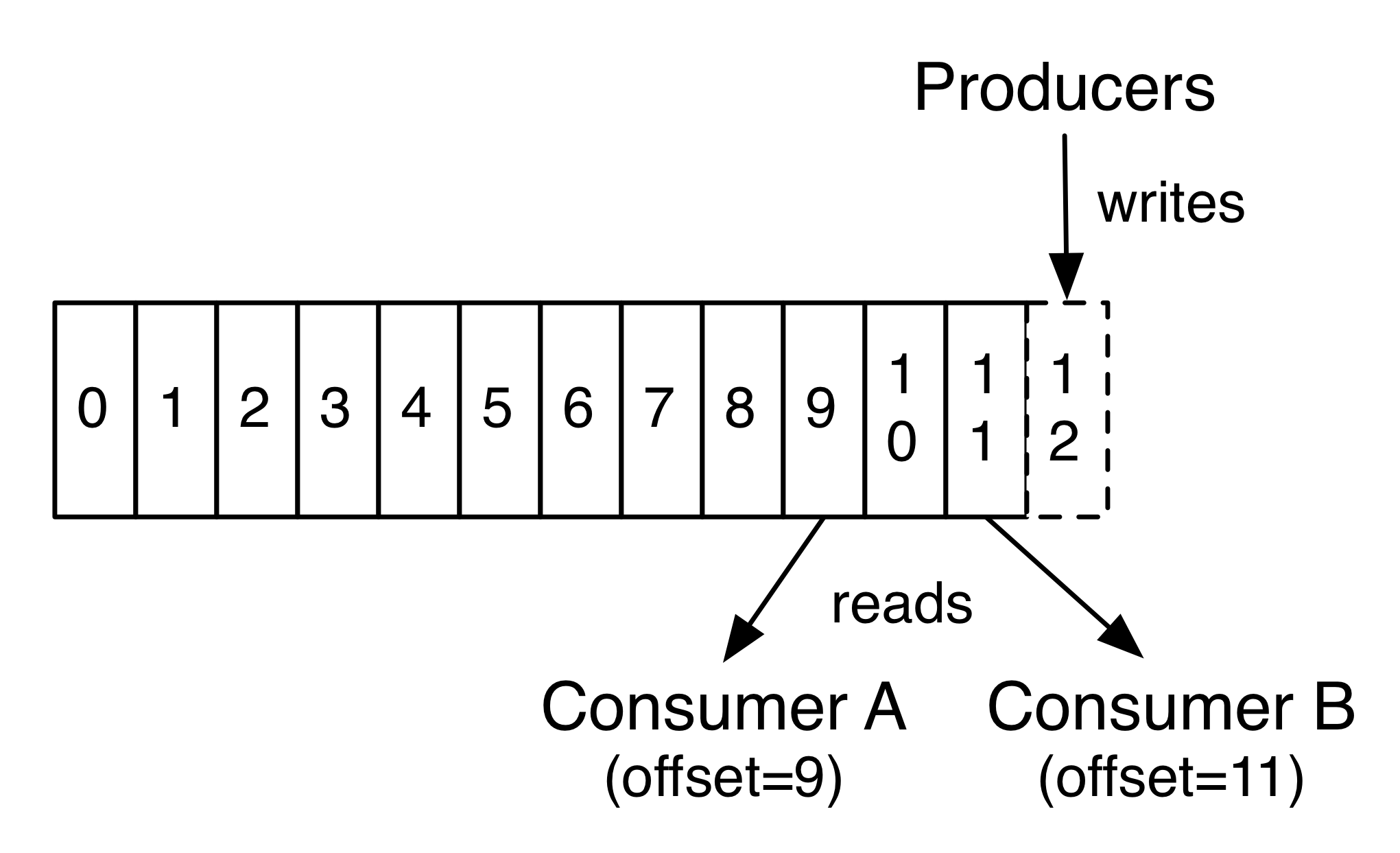
Components:
Kafka Broker : Kafka cluster typically consists of multiple brokers to maintain load balance. Kafka brokers are stateless, so they use ZooKeeper for maintaining their cluster state.
Producer : Producers publish data to the topics. Producers are the publisher of messages to one or more Kafka topics.
Consumer : Consumers read data from brokers. Consumers subscribes to one or more topics and consume published messages by pulling data from the brokers.
Topic : Stream of records. A topic is a category or feed name to which records are published.
Partition: For each topic, the Kafka cluster maintains a partitioned log. Each partition is an ordered, immutable sequence of records that is continually appended to—a structured commit log. The records in the partitions are each assigned a sequential id number called the offset that uniquely identifies each record within the partition.
Note: Kafka only provides a total order over records within a partition, not between different partitions in a topic.