Given strings s1
, s2
, and s3
, find whether s3
is formed by an interleaving of s1
and s2
.
An interleaving of two strings s
and t
is a configuration where they are divided into non-empty substrings such that:
s = s1 + s2 + ... + sn
t = t1 + t2 + ... + tm
|n - m| <= 1
- The interleaving is
s1 + t1 + s2 + t2 + s3 + t3 + ...
ort1 + s1 + t2 + s2 + t3 + s3 + ...
Note: a + b
is the concatenation of strings a
and b
.
Example 1:
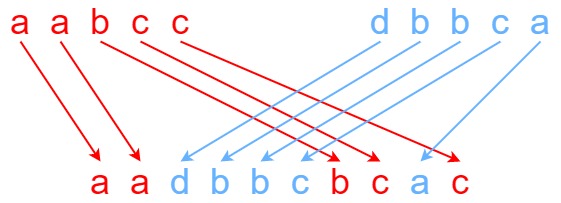
Input: s1 = "aabcc", s2 = "dbbca", s3 = "aadbbcbcac" Output: true
Example 2:
Input: s1 = "aabcc", s2 = "dbbca", s3 = "aadbbbaccc" Output: false
Example 3:
Input: s1 = "", s2 = "", s3 = "" Output: true
Constraints:
0 <= s1.length, s2.length <= 100
0 <= s3.length <= 200
s1
,s2
, ands3
consist of lowercase English letters.
Approach 3: Using 2D Dynamic Programming
Algorithm
The recursive approach discussed in above solution included a character from one of the strings or in the resultant interleaved string and called a recursive function to check whether the remaining portions of and can be interleaved to form the remaining portion of . In the current approach, we look at the same problem the other way around. Here, we include one character from or and check whether the resultant string formed so far by one particular interleaving of the the current prefix of and form a prefix of .
Thus, this approach relies on the fact that the in order to determine whether a substring of (upto index ), can be formed by interleaving strings and upto indices and respectively, solely depends on the characters of and upto indices and only and not on the characters coming afterwards.
To implement this method, we'll make use of a 2D boolean array . In this array implies if it is possible to obtain a substring of length which is a prefix of by some interleaving of prefixes of strings and having lengths and respectively. For filling in the entry of , we need to consider two cases:
The character just included i.e. either at index of or at index of doesn't match the character at index of , where . In this case, the resultant string formed using some interleaving of prefixes of and can never result in a prefix of length in . Thus, we enter at the character .
The character just included i.e. either at index of or at index of matches the character at index of , where . Now, if the character just included(say ) which matches the character at index of , is the character at index of , we need to keep at the last position in the resultant interleaved string formed so far. Thus, in order to use string and upto indices and to form a resultant string of length which is a prefix of , we need to ensure that the strings and upto indices and respectively obey the same property.
Similarly, if we just included the character of , which matches with the character of , we need to ensure that the strings and upto indices and also obey the same property to enter a at .
This can be made clear with the following example:
s1="aabcc"
s2="dbbca"
s3="aadbbcbcac"
public class Solution {
public boolean isInterleave(String s1, String s2, String s3) {
if (s3.length() != s1.length() + s2.length()) {
return false;
}
boolean dp[][] = new boolean[s1.length() + 1][s2.length() + 1];
for (int i = 0; i <= s1.length(); i++) {
for (int j = 0; j <= s2.length(); j++) {
if (i == 0 && j == 0) {
dp[i][j] = true;
} else if (i == 0) {
dp[i][j] = dp[i][j - 1] && s2.charAt(j - 1) == s3.charAt(i + j - 1);
} else if (j == 0) {
dp[i][j] = dp[i - 1][j] && s1.charAt(i - 1) == s3.charAt(i + j - 1);
} else {
dp[i][j] = (dp[i - 1][j] && s1.charAt(i - 1) == s3.charAt(i + j - 1))
|| (dp[i][j - 1] && s2.charAt(j - 1) == s3.charAt(i + j - 1));
}
}
}
return dp[s1.length()][s2.length()];
}
}
Complexity Analysis
Time complexity : . dp array of size is filled.
Space complexity : . 2D dp of size is required. and are the lengths of strings and respectively.
No comments:
Post a Comment