Given the head
of a linked list, remove the nth
node from the end of the list and return its head.
Follow up: Could you do this in one pass?
Example 1:
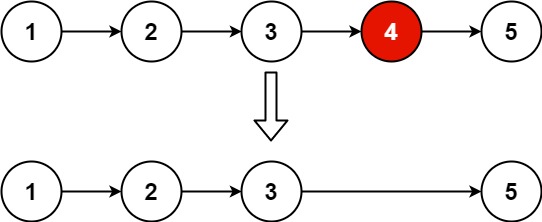
Input: head = [1,2,3,4,5], n = 2 Output: [1,2,3,5]
Example 2:
Input: head = [1], n = 1 Output: []
Example 3:
Input: head = [1,2], n = 1 Output: [1]
Solution:
public ListNode removeNthFromEnd(ListNode head, int n) {
if(head == null)
return head;
ListNode dummy = new ListNode(0);
dummy.next = head;
ListNode first, second;
first=second=dummy;
for(int i=0; i<n+1; i++) {
first=first.next;
}
while(first!=null){
first=first.next;
second=second.next;
}
// Remove nth node from end
second.next = second.next.next;
return dummy.next;
}
No comments:
Post a Comment